Retrieving JSON with AJAX
In this tutorial, we will retrieve some JSON data from external sources via AJAX using the XMLHttpRequest
object.
We will be using just plain JavaScript to get JSON from URL without jQuery.
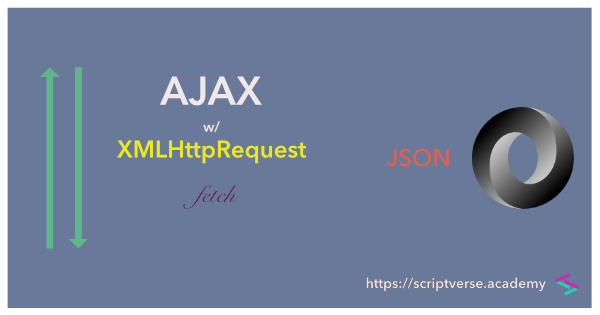
We first consider a simple JSON from http://ip.jsontest.com/
, which returns your IP address. It looks something like
{"ip": "182.75.39.2"}
We first create an instance of the XMLHttpRequest
object, say xhr
,
var xhr = new XMLHttpRequest();
and initialize the open
method by passing the GET
request as the first argument for retrieving JSON data from the url http://ip.jsontest.com/
, which is passed as the second argument. The last argument is a Boolean value: true
for asynchronous requests or false
for synchronous requests.
var xhr = new XMLHttpRequest();
xhr.open("GET", "http://ip.jsontest.com/", true);
Now after sending request to the server, we will want to do certain operations based on the response. Here, the onreadystatechange
event handler comes in handy, which is called everytime the readystatechange
event is fired, which in turn is fired every time the readyState
attribute of the XMLHttpRequest
object changes. The readyState
attribute gives the state of the XMLHttpRequest
client in numerical values from 0 to 4. The value is 4 when the fetch operation is complete. The other property of the XMLHttpRequest
object to consider is the status
property which returns the standard HTTP status codes. When a request is successful the status value is 200. We check for these two conditions when readyState
equals 4 and status
equals 200 and perform action on the response recieved. Since we have requested a non-XML data, the response data will be in responseText
property as a string. (For XML data, response data will be in responseXML
property). Now here is where the beginners often ask, how to parse the JSON object upon AJAX request success
? We parse this JSON string into JavaScript object using the JSON.parse() method and display some of its property values in the console. Here is the code.
var xhr = new XMLHttpRequest();
xhr.open("GET", "http://ip.jsontest.com/", true);
xhr.onreadystatechange = function() {
if(xhr.readyState == 4 && xhr.status == 200) {
var address = JSON.parse(xhr.responseText);
console.log("IP: ", address.ip);
}
}
Finally, we complete our request to the server via the send
method. Wrapping it all in a self-invoking function, it becomes
(
function(){
var xhr = new XMLHttpRequest();
xhr.open("GET", "http://ip.jsontest.com/", true);
xhr.onreadystatechange = function() {
if(xhr.readyState == 4 && xhr.status == 200) {
var address = JSON.parse(xhr.responseText);
console.log("IP: ", address.ip);
}
}
xhr.send();
}
)();
The response data displayed in the browser console will be
IP:
We will consider another JSON — a bit more complex than the above. The JSON will be fetched from https://jsonplaceholder.typicode.com/users, which is structured as follows.
[
{
"id": 1,
"name": "Leanne Graham",
"username": "Bret",
"email": "Sincere@april.biz",
"address": {
"street": "Kulas Light",
"suite": "Apt. 556",
"city": "Gwenborough",
"zipcode": "92998-3874",
"geo": {
"lat": "-37.3159",
"lng": "81.1496"
}
},
"phone": "1-770-736-8031 x56442",
"website": "hildegard.org",
"company": {
"name": "Romaguera-Crona",
"catchPhrase": "Multi-layered client-server neural-net",
"bs": "harness real-time e-markets"
}
},
{
"id": 2,
"name": "Ervin Howell",
"username": "Antonette",
"email": "Shanna@melissa.tv",
"address": {
"street": "Victor Plains",
"suite": "Suite 879",
"city": "Wisokyburgh",
"zipcode": "90566-7771",
"geo": {
"lat": "-43.9509",
"lng": "-34.4618"
}
},
"phone": "010-692-6593 x09125",
"website": "anastasia.net",
"company": {
"name": "Deckow-Crist",
"catchPhrase": "Proactive didactic contingency",
"bs": "synergize scalable supply-chains"
}
}
]
We will fetch it via AJAX using the XMLHttpRequest
object and display the names as list inside the <ul/>
element with id=users
. This also shows you how to display JSON data in HTML using AJAX.
<ul id="users"></ul>
The code for this is as follows:
(
function(){
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://jsonplaceholder.typicode.com/users", true);
xhr.onreadystatechange = function() {
if(xhr.readyState == 4 && xhr.status == 200) {
var users = JSON.parse(xhr.responseText);
let names = "";
users.map((itm,idx) => {
names += "<li>" + itm.name + "</li>";
}, this);
document.getElementById('users').innerHTML = names;
}
}
xhr.send();
}
)();
After fetching, the names are populated inside the <ul/>
element with id=users
as
Notes
-
More on values of
readyState
property of theXMLHttpRequest
object can be read on the following link https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/readyState.