C Program: Generate a Random Matrix
To generate a random matrix, we need to generate random numbers.
In this C program, we will be making use of the rand()
function under the <stdlib.h>
header file. The rand()
function generates numbers from 0
to RAND_MAX
, the value of which is system dependent.
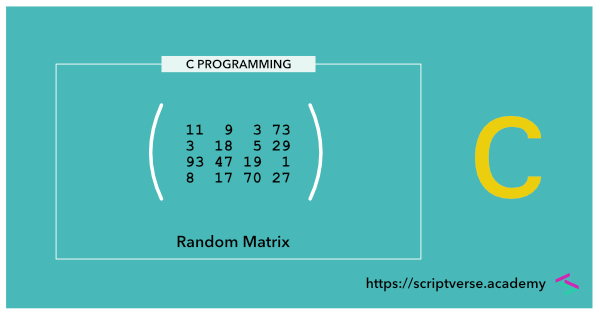
You can make a quick check of the RAND_MAX
value in your system.
printf("%d", RAND_MAX);
To generate random numbers from 0 to 99 we need to take rand()
modulo 100, or rand() % 100
.
The below program generates a square matrix of random numbers from 0 to 99. We make use of two for
loops: one to loop over the rows and the other to loop over the columns. Notice the space in the statement printf("%d ", rand()%100);
#include <stdio.h>
#include <stdlib.h>
int main() {
unsigned short i, j, n;
printf("Enter the dimension of the matrix: ");
scanf("%hu", &n);
for(i = 0; i < n;i++) {
for(j = 0; j < n;j++) {
printf("%d ", rand()%100);
}
printf("\n");
}
return 0;
}
We can modify the above code to accept the row and column dimensions separately to generate an $m$ x $n$ matrix ($m$ rows and $n$ columns).
#include <stdio.h>
#include <stdlib.h>
int main() {
unsigned short i, j, m, n;
printf("Enter the row dimension of the matrix: ");
scanf("%hu", &m);
printf("Enter the column dimension of the matrix: ");
scanf("%hu", &n);
for(i = 0; i < m;i++) {
for(j = 0; j < n;j++) {
printf("%d ", rand()%100);
}
printf("\n");
}
return 0;
}
We run the program for $3 \times 4$ matrix.
$ ./a.out
Enter the row dimension of the matrix: 3
Enter the column dimension of the matrix: 4
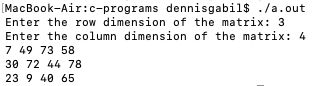