C Program: Find the Largest/Greatest/Maximum of Three Numbers
In this tutorial, we will write a simple C program to find largest of three given numbers.
Consider the three numbers a
, b
and c
.
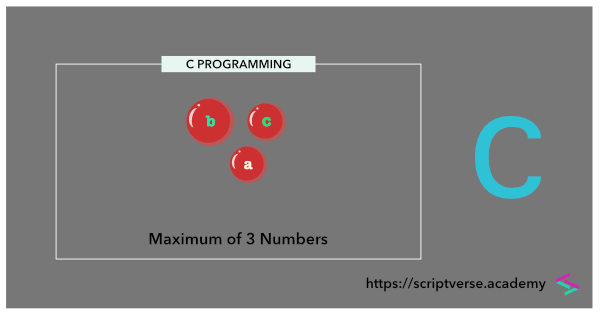
Below is a simple algorithm for finding the largest among them:
Step 1: Start
Step 2: Declare three integer variables a, b, c
Step 3: If a is greater than b,
If a is greater than c,
Print "a is largest"
Else
Print "c is largest"
Else
If b is greater than c,
Print "b is largest"
Else
Print "c is largest"
Step 4: Stop
The above algorithm is implemented into a C program below.
#include <stdio.h>
int main() {
int a, b, c;
printf("Enter the first number: ");
scanf("%d", &a);
printf("Enter the second number: ");
scanf("%d", &b);
printf("Enter the third number: ");
scanf("%d", &c);
if (a >= b) {
if (a >= c)
printf("The largest number is %d.\n", a);
else
printf("The largest number is %d.\n", c);
}
else {
if (b >= c)
printf("The largest number is %d.\n", b);
else
printf("The largest number is %d.\n", c);
}
return 0;
}
On executing the above program, the prompt to enter numbers will appear three times as follows:
$ ./a.out
Enter the first number:
Enter the second number:
Enter the third number:
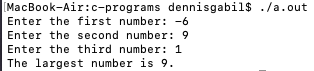
The above program has several nested if
statements. Here is a much simpler and shorter program, based on an algorithm given here, to achieve the same.
#include <stdio.h>
int main() {
int a, b, c, max;
printf("Enter the first number: ");
scanf("%d", &a);
printf("Enter the second number: ");
scanf("%d", &b);
printf("Enter the third number: ");
scanf("%d", &c);
max = a;
if(b > max) {
max = b;
}
if(c > max) {
max = c;
}
printf("The largest number is %d.\n", max);
return 0;
}