Array filter() Method
The JavaScript filter()
method returns a new array consisting of all the elements that meet the condition set in its callback function.
The syntax is as follows
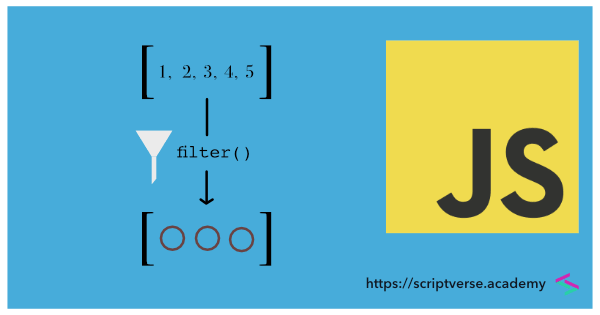
array.filter(callback(value,index,arr), thisValue)
The callback()
function has three arguments as follows
value
index
arr
The second parameter thisValue
is the value to be used as this
in the callback function.
Consider the array
[1, 2.718, Math.PI, 'hello', 4, 5]
where PI
is a property of the JavaScript Math
object, which has the value of π upto fifteen decimal places. In the callback function, using the Number.isInteger()
method, we set the condition for only the integer values of the array to pass through
var integer = [1, 2.718, Math.PI, 4, 'hello', 5].filter(function(value){
return Number.isInteger(value);
});
console.log(integer);
We can also rewrite the above script in the newer ES6 arrow function notation.
var integer = [1, 2.718, Math.PI, 4, 'hello', 5].filter(value => Number.isInteger(value));
console.log(integer);
Since the elements 2.718
, Math.PI
and 'hello'
are not integers, they do not pass the test and only the remaining elements gets returned as the elements of a new array and gets printed into the console
[1, 4, 5]
undefined
and null
Elements
The callback method inside filter()
is called even for undefined
and null
elements. In this script, you will find that although both undefined
and null
did not qualify the condition set in the callback, their indices were printed into the browser's console.
var integer = [1, 4, undefined, null].filter((value, idx) => {
console.log(idx);
return Number.isInteger(value);
});
console.log(integer);
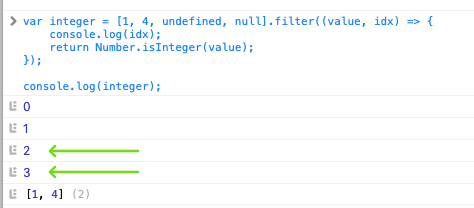
Deleted Elements
Unlike for undefined
and null
elements, the callback method inside the filter()
method is not invoked for deleted array elements. In the below script, the 'hello' element is deleted. Hence the callback is not called for that particular element.
var integer = [1, 2.718, Math.PI, 4, 'hello', 5];
delete integer[4]; // delete the element 'hello'
integer.filter((value, idx) => {
console.log(idx);
return Number.isInteger(value);
});
You can see that the index of the deleted 'hello' element is not printed.
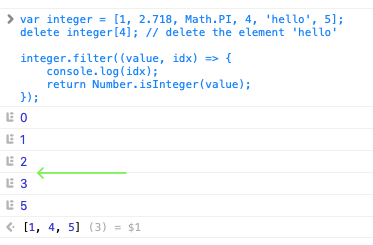
The thisValue
Parameter
We can pass a value into the anonymous callback function which can then be used as this
inside it. Here we make use the same array defined above and pass 'hello'
as the second argument to the .filter()
method, which is then used as this
inside the anonymous callback function
var h = [1, 2.718, Math.PI, 4, 'hello', 5].filter(function(value){
return value == this;
}, 'hello');
console.log(h);
Of course, there is only one element which passes the condition set by the callback here.
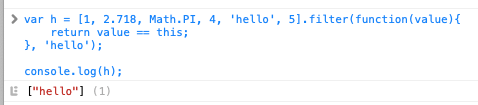
Search Array
The filter()
method is particularly useful in dropdowns built with select option
or ul li
elements where there is an input search box.
Here we will not do a dropdown, but a simple input box which filters out matching elements from the following array based on the search string.
['Singapore', 'Tokyo', 'Beijing', 'Kuala Lumpur', 'Hong Kong'];
The search box is a simple <input/>
element
<input type="text" id="search"/>
And here is the script which makes use of the filter()
method to search the given array
const cities = ['Singapore', 'Tokyo', 'Beijing', 'Kuala Lumpur', 'Hong Kong'];
document.getElementById('search').addEventListener('input', function(e) {
const filterItems = (arr, query) => {
return arr.filter(el => el.toLowerCase().indexOf(query.toLowerCase()) > -1);
};
const filtered = filterItems(cities, e.target.value);
document.getElementById('results').innerHTML = filtered.join(", ");
});
Try it out: