MongoDB Tutorial
MongoDB has become one of the most popular cross-platform document-oriented NoSQL databases. It was first released on February 11, 2009, and is currently being developed and maintained by MongoDB Inc (formerly known as 10gen).
MongoDB was started by Dwight Merriman, Eliot Horowitz and Kevin Ryan in 2007, when existing databases posed serious set-backs in terms of scalability and agility to their then Internet advertising company DoubleClick (later brought by Google for $3.1 billion), which was serving about 400,000 ads per second.
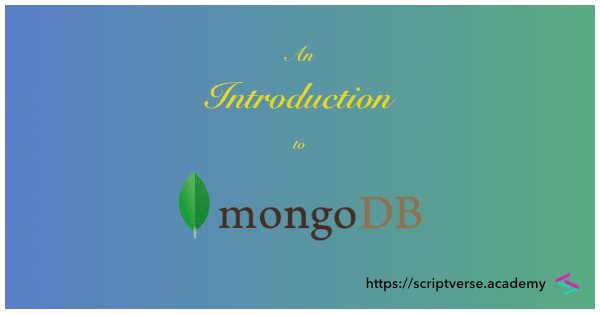
MongoDB was written in C++, Go, JavaScript and Python.
Sci-fi comic book buffs will be familiar with the name Mongo from Flash Gordon comics.
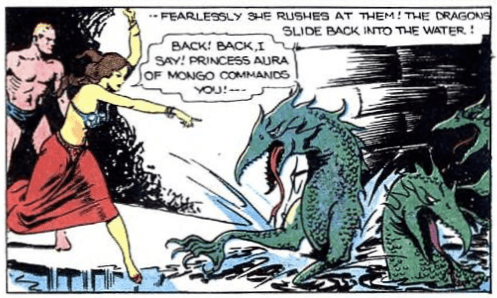
In the following list, we outline some of the prominent features of MongoDB.
-
BSON. A Binary JSON format to represent documents which uses the
_id
field as its primary key. - Flexible Schema. Two documents in a collection need not be of identical schema.
- Indexing. Facility for creating single or multiple indexes for quicker query performance.
- Ad-hoc Queries. Support for queries updated in real time, which were unknown during structuring the datatbase.
- Sharding. Achieving horizontal scalibility by distributing data across multiple machines, known as sharding.
- Aggregation. Returning computed results of processed data records.
- MapReduce. A paradigm in data-processing where large volumes of data is condensed into useful aggregated result.
- GridFS. A GridFS specification to store files exceeding 16 MB.
- Replication. Ensure data availability with group of Mongo Daemon instances that maintain the same data set for cases of primary server fails.
The corporations and companies which use MongoDB include Google, Adobe, Facebook, Nokia, SAP, KPMG, etc. A list is maintained here.
Installation
There is a dedicated page in the official documentation for installing MongoDB on Linux, macOS and Windows here.
In the below sections, we present a list of essential commands in a nutshell to perform basic CRUD operations in MongoDB.
Create, Show & Delete Database
Unlike in SQL, there is no magical CREATE DATABASE
statement to create a database in MongoDB explicitly. Creating a database in MongoDB involves switching to it first using the use
statement and inserting at least one document to a collection in it.
To create a database called books
, we first switch to it as follows
$ use books
This however does not create the database yet. You can type the following command to list all the available databases
$ show dbs
and you will find that only the default test
database is listed; the books
database which is supposed to be "created" is not there in the list yet. This is because, for a database to be created in MongoDB, you need to save at least one document in a collection.
Now if you need to delete some unwanted database, you can make use of the dropDatabase() method after switching to it as shown here
$ use books
$ db.dropDatabase()
Create, Show & Delete Collections
In MongoDB, each database consists of collections, and each collection stores records (actually known as "documents"). Collections are analogous to tables in relational databases. To create a collection, we make use of the createCollection() method.
Below we create a new collection called comics
belonging to the books
database
$ use books
$ db.createCollection('comics')
To list all collections in a books
database, you can type the below command
$ use books
$ show collections
If you want to remove a collection belonging to some database, chain the drop() method to it as shown here
$ use books
$ db.comics.drop()
Create, Query, Update & Delete Documents
It is high time we add some record to our comics
collection inside the books
database. We add a document consisting of the title
, author
and year
fields with some values.
$ use books
$ db.comics.insert({
title: 'Flash Gordon and the Witch Queen of Mongo',
author: 'Alex Raymond',
year: 1936
})
All documents inside a collection can be listed chaining the find()
method to it
$ use books
$ db.comics.find()
Now if you look closely at the result, you will find one _id
field automatically indexed. This _id
field holds an ObjectId and is the default primary key for a MongoDB document.
If you desire a formatted output, chain the pretty()
method to the find()
method.
$ db.comics.find().pretty()
To query a particular document with some field having a specific value, pass the key-value pair as an object to the find()
method. Here we query a document with the title
field having the value Flash Gordon and the Witch Queen of Mongo
$ db.comics.find({
"title": 'Flash Gordon and the Witch Queen of Mongo'
})
Suppose you wish to make some changes to the document and update it. Say, you want to change and update its title and year.
$ db.comics.update({"_id":ObjectId("5d43eb866f28a586597cccad")},{
$set:{
'title':'Flash Gordon and the Ape Men of Mor',
'year': 1942
}
})
To remove all documents/records with the title field having the value Flash Gordon and the Ape Men of Mor
, run the command
$ db.comics.remove({"title": "Flash Gordon and the Ape Men of Mor"})
and it will remove all documents with the title Flash Gordon and the Ape Men of Mor
in the comics
collection.
If you wish to remove just the first occurrence of the document in a collection with the title Flash Gordon and the Ape Men of Mor
, pass along 1
as the second parameter.
$ db.comics.remove({"title": "Flash Gordon and the Ape Men of Mor"}, 1)
If you wish to remove all documents in a collection, you need to pass a pair of empty parentheses to the remove()
method.
$ db.comics.remove({})
Notes
- MongoDB, Inc. offers online courses on MongoDB at MongoDB University for free.