Node.js: Introduction/ Setting Up an HTTP Web Server
Node.js is an open-source cross-platform JavaScript runtime environment built on Chrome's V8 JavaScript engine.
It has an event-driven architecture coupled with I/O methods, all asynchronous and accepting callback functions, which in turn provides a non-blocking flow. Node.js is thus suitable for building highly scalable network applications.
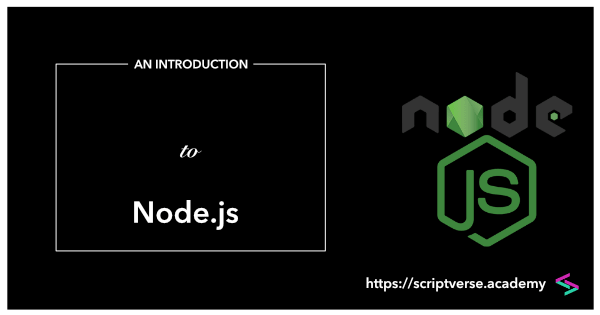
To install Node.js via the package manager, follow the link below. It contains instructions for supported operating systems.
https://nodejs.org/en/download/package-manager/
After successful installation of Node.js, you can check its version typing the below command in the terminal.
node -v
It will print out the version number, something like
v10.15.3
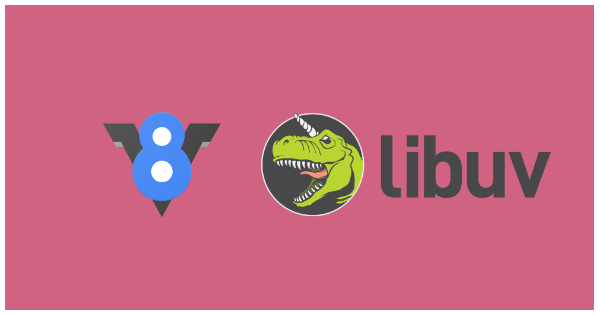
Node.js uses the V8 JavaScript engine, which was originally built for Google Chrome. Written in C++, V8 does not interpret a JavaScript source code, it compiles it into native machine code. For handling asynchronous events, Node.js uses another cross-platform C library called Libuv.
Creatng Your First Node.js Web Server
In this section, we will be creating our very first Node.js HTTP web server to render and display a text. Create a directory, say, /node-programs
. Inside it, create an empty JS file called server.js
and copy-paste the following code.
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World\n');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Run the HTTP web server we just created, typing the command
node server.js
Inside the Node.js console, there will be a message about the host and port on which the server is running. It will be http://localhost:3000/.
http://localhost:3000/
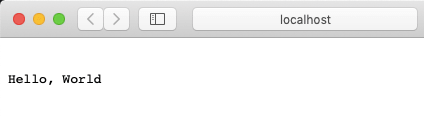