C Program: Length/Size of an Array
In our C program below, we will count the number of elements in various arrays of different data types.
The array c
is of type char
and contains six characters; the array n
is of int
type and contains nine integers; the array f
has two elements of type float; and the array d
of type double
has three elements.
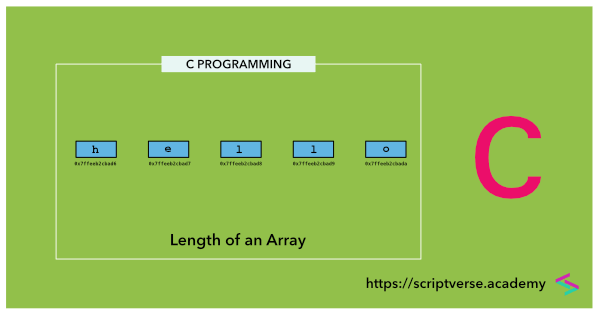
We make use of the sizeof()
unary operator which returns the size of a data type in bytes. So, sizeof(char)
will return 1, sizeof(int)
will return 2, sizeof(float)
will return 4 and sizeof(double)
will return 8. So the total size an array occupies will be the number of its elements × size of data type. That means, if we we divide the size of array with its data type, we get the number of elements in it. This is what we have implemented in the below C program.
#include <stdio.h>
int main() {
char c[] = "hello";
int n[] = {-4, -3, -2, -1, 0, 1, 2, 3, 4};
float f[] = {3.14, 2.71};
double d[] = {3.14, 2.71, 1.61};
printf("Size of the character array is: %lu", sizeof(c)/sizeof(char));
printf("\n");
printf("Size of the integer array is: %lu", sizeof(n)/sizeof(int));
printf("\n");
printf("Size of the float array is: %lu", sizeof(f)/sizeof(float));
printf("\n");
printf("Size of the double array is: %lu", sizeof(d)/sizeof(double));
printf("\n");
return 0;
}
We can also find the length of an array by using pointer arithmetic. A little note on the difference between &n and &n[0] and why we have picked the former. If you check their values using printf()
, they both will show the same address. However, upon incrementing, (&n + 1) points to the next memory block after the whole array while (&n[0] + 1) points to n[1], the next element in the array.
#include <stdio.h>
int main() {
int n[] = {-4, -3, -2, -1, 0, 1, 2, 3, 4};
unsigned short size = *(&n + 1) - n;
printf("Size of the integer array is: %hu", size);
return 0;
}