C Program: Sum of First N Natural Numbers
In this tutorial, we will be writing C programs to find the sum of first $n$ natural numbers, i.e., $1 + 2 + 3 + 4 + ... + n$.
In our first program below, we make use of the for
loop to repeatedly add the consecutive terms to get the desired sum. Also, as there will not be any negative values involving here, we define the required variables as of unsigned
types.
#include <stdio.h>
int main() {
unsigned short int i, n;
unsigned long int sum = 0;
printf("Enter a natural number: ");
scanf("%hu", &n);
for(i = 1; i <= n; i++) {
sum += i;
}
printf("The sum of first %hu natural numbers is %lu", n, sum);
return 0;
}
We can also get the above sum using any of the while
or do-while
loops.
Using the Formula $\frac{n(n+1)}{2}$
But there exists a much better way to get that sum without using any loops. We will make use of the well-known formula that we learned in our basic Sequences/Series lessons involving Arithmetic Progression: $1 + 2 + 3 + 4 + ... + n$ = $\frac{n(n+1)}{2}$
The above formula is connected to a well-known legend about the childhood of Carl Friedrich Gauss (also known as "Princeps Mathematicorum"), where he added the numbers from 1 upto 100 in a matter of seconds.
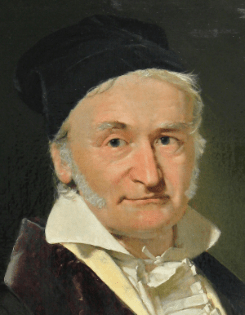
The C program below makes use of the $\frac{n(n+1)}{2}$ formula instead of adding terms repeatedly using loops.
#include <stdio.h>
int main() {
unsigned short int n;
unsigned long int sum = 0;
printf("Enter a natural number: ");
scanf("%hu", &n);
sum = n*(n+1)/2;
printf("The sum of first %hu natural numbers is %lu", n, sum);
return 0;
}