C Program: Quadratic Equation
A second order polynomial equation of type $ax^{2} + bx + c = 0$, where $x$ is a variable and $a \ne 0$ is known as a Quadratic Equation.
Here, we will be writing a C program to find the roots of a quadratic equation $ax^{2} + bx + c = 0$.
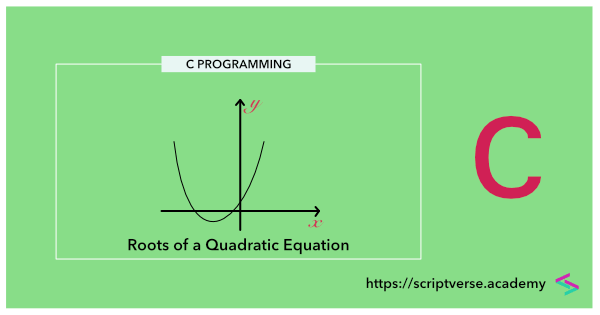
By the Fundamental Theorem of Algebra, a quadratic equation has two roots. These roots are given by
$x = \frac{-b \pm \sqrt{b^{2} - 4ac}}{2a}$
This is also known as the Quadratic Formula.
The expression $b^{2} - 4ac$ is known as the discriminant of the quadratic equation. The nature of the roots depend heavily on it. From the quadratic formula, we can make out that if
- $b^{2} - 4ac > 0$, the roots are real and unequal
- $b^{2} - 4ac = 0$, the roots are real and equal
- $b^{2} - 4ac < 0$, the roots are imaginary
In the below C program, the roots are computed inside the if
, else if
and else
blocks, each depending on whether the discriminant is $\gt 0$, $= 0$ and $\lt 0$. The else
part of the loop computes the real and imaginary parts of the roots separately. Since the discriminant is $\lt 0$ (negative), the minus sign is prepended to it to make it positive; for if not, the sqrt()
function will return nan
("Not a Number"). The variables x1
and x2
are assigned the computed values of the roots based on the quadratic formula.
The <math.h>
library is imported to make use of the pow()
and sqrt()
functions.
#include <stdio.h>
#include <math.h>
int main() {
float a, b, c, discriminant, x1, x2, r, i;
printf("coefficient of x^2: ");
scanf("%f", &a);
printf("coefficient of x: ");
scanf("%f", &b);
printf("constant term: ");
scanf("%f", &c);
discriminant = pow(b,2) - 4*a*c;
if(discriminant > 0) {
x1 = (-b + sqrt(discriminant))/(2*a);
x2 = (-b - sqrt(discriminant))/(2*a);
printf("x1 = %.2f \n", x1);
printf("x2 = %.2f \n", x2);
} else if (discriminant == 0) {
x1 = -b/(2*a);
x2 = -b/(2*a);
printf("x1 = %.2f \n", x1);
printf("x2 = %.2f \n", x2);
} else {
r = -b/(2*a);
i = sqrt(-discriminant)/(2*a);
printf("x1 = %.2f +i %.2f \n", r, i);
printf("x2 = %.2f -i %.2f \n", r, i);
}
return 0;
}
We take a quadratic equation straight out of the classic Hall & Knight's text Elementary Algebra1, which is as follows:
On deducing, the roots are found to be $\frac{15 + \sqrt{5}}{10}$ and <$\frac{15 - \sqrt{5}}{10}$ respectively. Substituting for $\sqrt{5} \approx 2.236$, we get the approximate values of the roots as $1.7236$ and $1.2764$.
Running our program for the above equation, we get:
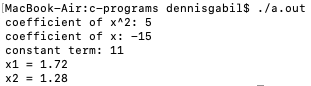
Notes
- 1. H. S. Hall & S. R. Knight, Elementary Algebra. London: Macmillan & Co., Ltd., 1896. Chapter XXVI: Quadratic Equations, p. 241, Ex. 1.