C Program: Length of a String
There already exists a built-in function called strlen()
to compute the length of a string, and is available under the <string.h>
header library. The given string needs to be passed as an argument to strlen()
; it then returns its length.
The program below illustrates the use of strlen()
to find the length of a given string. We use scanf("%[^\n]")
to read the input string instead of the usual scanf("%s")
. The difference is, while scanf("%s")
stops reading characters on encounter of first blank space, the conversion specifier scanf("%[^\n]")
reads them like any normal character.
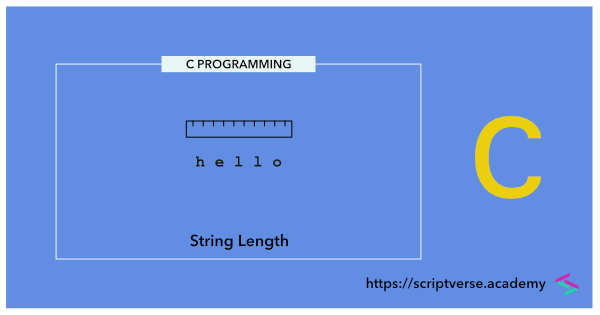
NOTE: As the gets()
function is already deprecated, we will not be using it anymore to read string inputs.
Also, the strlen()
function returns an integer of type unsigned long
, hence the use of the format specifier %lu
in the last printf()
statement.
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
printf("Enter a string: ");
scanf("%[^\n]", str);
printf("The length of the entered string is %lu \n", strlen(str));
return 0;
}
Length of String Without strlen()
We can also find the length of a given string without the use of the strlen()
function. The while
loop loops through each character of the string till the \0
or null character is reached, indicating the end of the string.
#include <stdio.h>
int main() {
char str[100];
unsigned short count = 0;
printf("Enter a string: ");
scanf("%[^\n]", str);
while (str[count] != '\0')
count++;
printf("The length of the entered string is %u: ", count);
return 0;
}